可视化
数据分析与数据科学项目中常用于数据可视化的Python包
- Matplotlib
- Seaborn
- pandas
- Bokeh
- Plotly
- Vispy
- Vega
- gega-lite
Matplotlib可视化
- 绘图API:pyplot,在Jupyte Notebook中,通常用pyplot进行可视化,本书采用的是matplotlib.pyplot。
- 集成库:pylab,pylab是Matplotlib和SciPy、NumPy的集成库。
- matplotlib的画图方式进一步分为两种:inline和notebook
- inline为静态绘图,且嵌入到Jupyter Notebook中
- notebook为交互式图,在Jupyter Notebook中中只显示一行输出代码
1 2 3 4 5 6 7
| import matplotlib.pyplot as plt %matplotlib inline import pandas as pd women = pd.read_csv('women.csv') women = pd.read_csv('women.csv',index_col =0) plt.plot(women["height"], women["weight"]) plt.show()
|
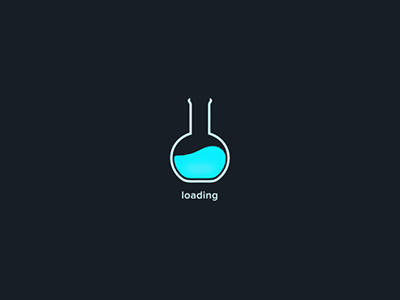
1 2 3 4
| import numpy as np t=np.arange(0.,4.,0.1) plt.plot(t,t,t,t+2,t,t**2,t,t+8) plt.show()
|
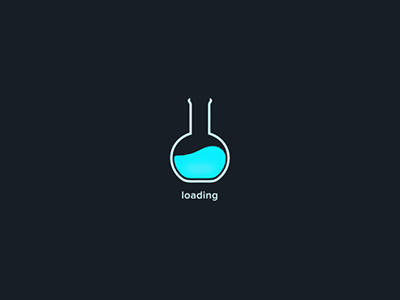
改变图的属性
1 2
| plt.plot(women["height"], women["weight"],"o") plt.show()
|
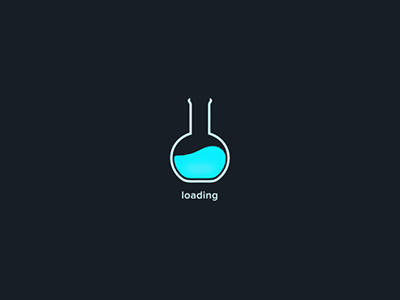
1 2
| plt.plot(women["height"], women["weight"],"g--") plt.show()
|
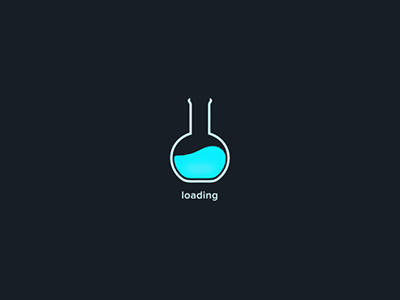
1 2 3 4 5 6
| plt.rcParams['font.family']="SimHei" plt.plot(women["height"], women["weight"],"g--") plt.title("此处为图名") plt.xlabel("x轴的名称") plt.ylabel("y轴的名称") plt.show()
|
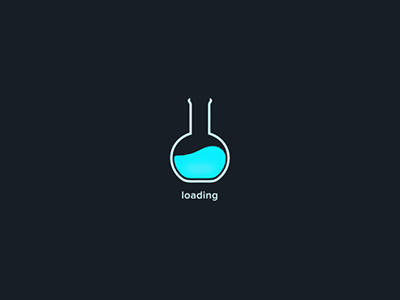
改变图的类型
1 2 3 4 5 6 7 8
| import matplotlib.pyplot as plt plt.bar([1,3,5,7,9],[5,2,7,8,2], label="Example one") plt.bar([2,4,6,8,10],[8,6,2,5,6], label="Example two", color='g') plt.legend() plt.xlabel('bar number') plt.ylabel('bar height') plt.title('Epic Graph\nAnother Line! Whoa') plt.show()
|
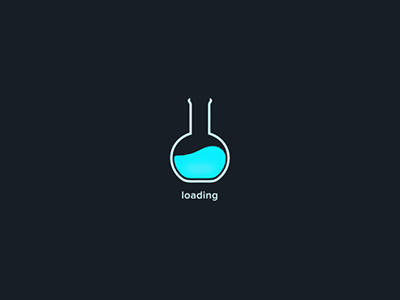
1 2 3 4 5 6 7
| import matplotlib.pyplot as plt population_ages = [22,55,62,45,21,22,34,42,42,4,99,102,110,120,121,122,130,111,115,112,80,75,65,54,44,43,42,48] plt.hist(population_ages, rwidth=0.8) plt.xlabel('x') plt.ylabel('y') plt.title('Interesting Graph\nCheck it out') plt.show()
|
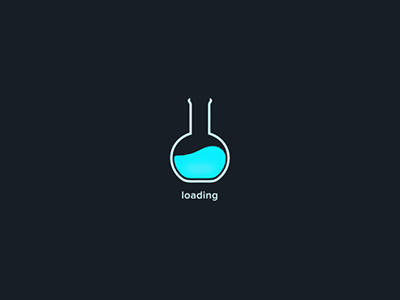
1 2 3 4 5 6 7 8 9
| import matplotlib.pyplot as plt x = [1,2,3,4,5,6,7,8] y = [5,2,4,2,1,4,5,2] plt.scatter(x,y, label='skitscat', color='k', s=25, marker="o") plt.xlabel('x') plt.ylabel('y') plt.title('Interesting Graph\nCheck it out') plt.legend() plt.show()
|
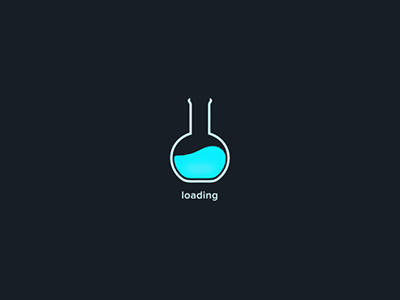
改变图的坐标轴的取值范围
- 横坐标分别定义:采用plt.xlim()或plt.ylim()
1 2 3 4 5 6 7 8
| import matplotlib.pyplot as plt import numpy as np %matplotlib inline x=np.linspace(0,10,100) plt.plot(x,np.sin(x)) plt.xlim(11,-2) plt.ylim(2.2,-1.3) plt.show()
|
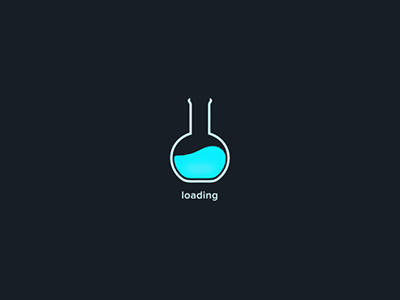
1 2 3 4 5 6 7
| import matplotlib.pyplot as plt import numpy as np %matplotlib inline x=np.linspace(0,10,100) plt.plot(x,np.sin(x)) plt.axis([-1,21,-1.6,1.6]) plt.show()
|
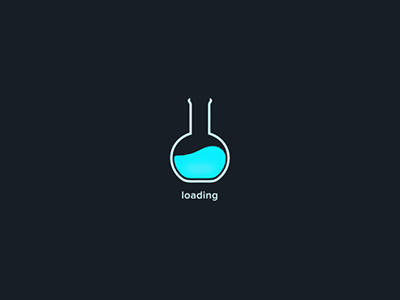
1 2 3 4
| plt.plot(x,np.sin(x)) plt.axis([-1,21,-1.6,1.6]) plt.axis("equal")
|
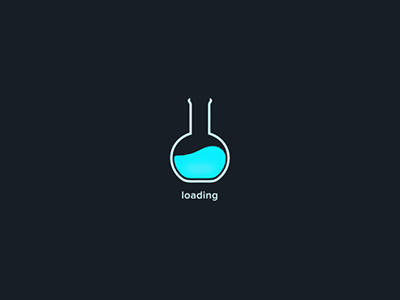
1 2 3 4 5 6 7 8
| import matplotlib.pyplot as plt import numpy as np %matplotlib inline x=np.linspace(0,10,100) plt.plot(x,np.sin(x)) plt.axis([-1,21,-1.6,1.6]) plt.axis("tight") plt.show()
|
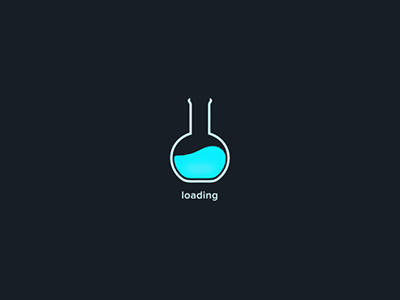
同坐标画两个图
1 2 3 4 5
| plt.plot(x,np.sin(x),label="sin(x)") plt.plot(x,np.cos(x),label="cos(x)") plt.axis("equal") plt.legend() plt.show()
|
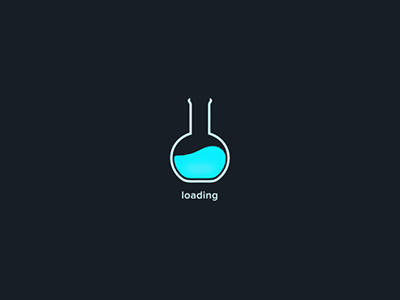
多图显示
1 2 3 4 5 6 7
| plt.subplot(2,3,5) plt.plot(x,np.sin(x)) plt.subplot(2,3,3) plt.plot(x,np.tan(x)) plt.subplot(2,3,1) plt.plot(x,np.cos(x)) plt.show()
|
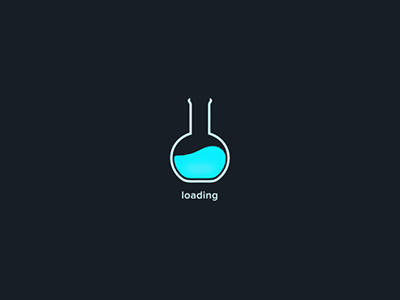
图的保存
1 2
| plt.plot(x,np.cos(x)) plt.savefig("1.png")
|